Refuse/Recycling Collection Alerts via Telegram
I use a python script to fetch my local council refuse collection web page to send me alerts in Telegram of upcoming collection days. I run this in 2 modes:
- weekly on a Sunday to give me a 7 day look ahead
- daily to alert me the day before the collection
The script sends a message to a Telegram bot - in telegram I subscribe to the bot and see the alerts when they come in.
How does it work?
It's a reasonably complicated script - and has to be tailored to a particular website. If I look at this site: https://bridgend.kier.co.uk/property/100100486073. (This is a random street near my Mums house), the key is to look at the page source and these two HTML table rows: [I have removed some data for brevity]
<tr class="service-id-76 task-id-45">
<td class="service-name"></td>
<td class="schedule">
<span class="table-label">Schedule</span>
<div>
Friday every week
</div>
</td>
<td class="last-service">
<span class="table-label">Last Service</span> 18/11/2022
</td>
<td class="next-service">
<span class="table-label">Next Service</span> 25/11/2022
</td>
</tr>
<tr class="service-id-74 task-id-43">
<td class="service-name">
<a title="PROPERTY_SERVICE_TOOLTIP" class="toggle-events collapsed" data-toggle="collapse" href="#serviceEvents74" aria-expanded="false" aria-controls="serviceTaskEvents43">Refuse Sacks collection</a>
</td>
<td class="schedule">
<span class="table-label">Schedule</span>
<div>
Friday every other week
</div>
</td>
<td class="last-service">
<span class="table-label">Last Service</span> 11/11/2022
</td>
<td class="next-service">
<span class="table-label">Next Service</span> 25/11/2022
</td>
</tr>
</tr>
So the row with class="service-id-76 task-id-45" is for Recycling and row with class="service-id-74 task-id-43" is normal refuse.
Using Pytthon with requests and BeautifulSoup you can fetch the page:
import requests
response = requests.request("GET","https://bridgend.kier.co.uk/property/100100486073")
and with BeautifulSoup
from bs4 import BeautifulSoup
soup = BeautifulSoup(requests, 'html.parser')
for rc in soup.find_all('tr',{'class':type}):
x = rc.find("td",class_="next-service").text
next_collection = x.replace("Next Service","").strip()
Where type = "service-id-76 task-id-45" or "service-id-74 task-id-43"
This gives you the Next Service date for the Recycling and Refuse collection. In my script I compare these dates to the current date and message accordingly.
Let me know if you want to see the full script or help with your local council website. My local site in Wiltshire has a month ahead calendar - so I load this into a database and then my daily/weekly scripts simply pull from the local database.
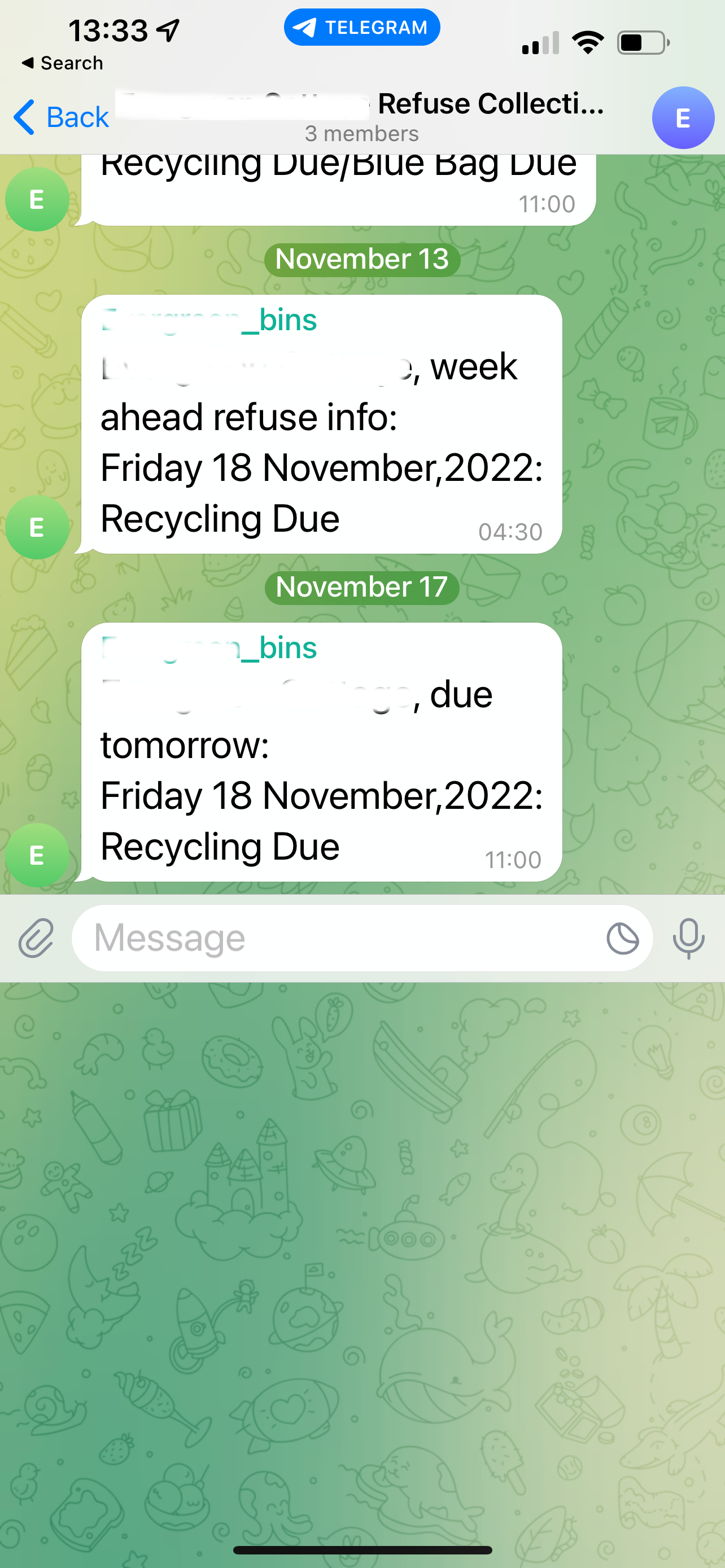
Comments