Blink Camera Movement as a Dashboard
Blink cameras are excellent at recording motion detection clips and notifying you via their mobile app - but what if you want to record motion detection in external apps?
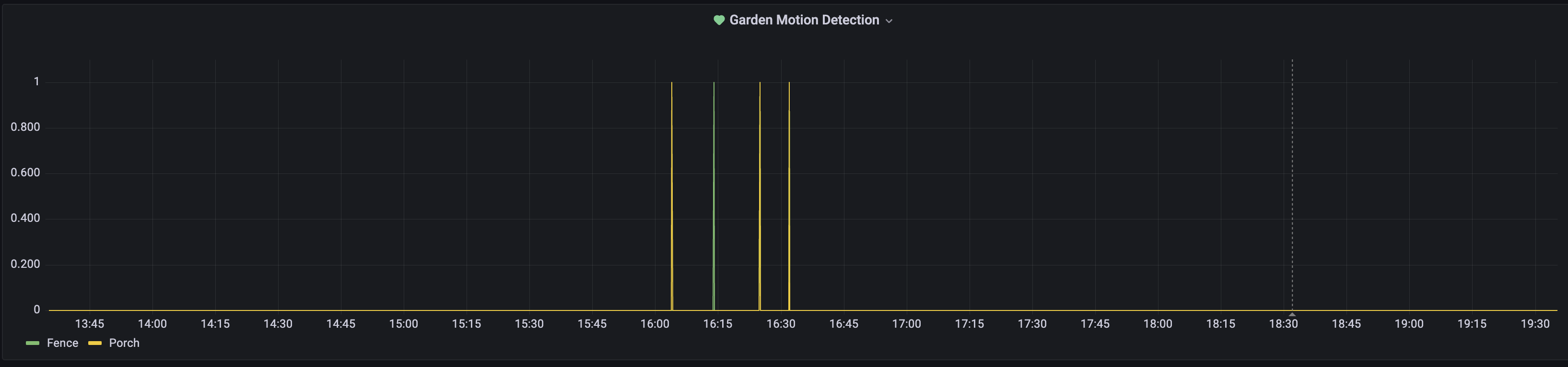
I use IFFFT and my own AWS instance running a python/flask script, influxDB and Grafana to record movement and send alert emails if no movement is detected in for 2 hours.
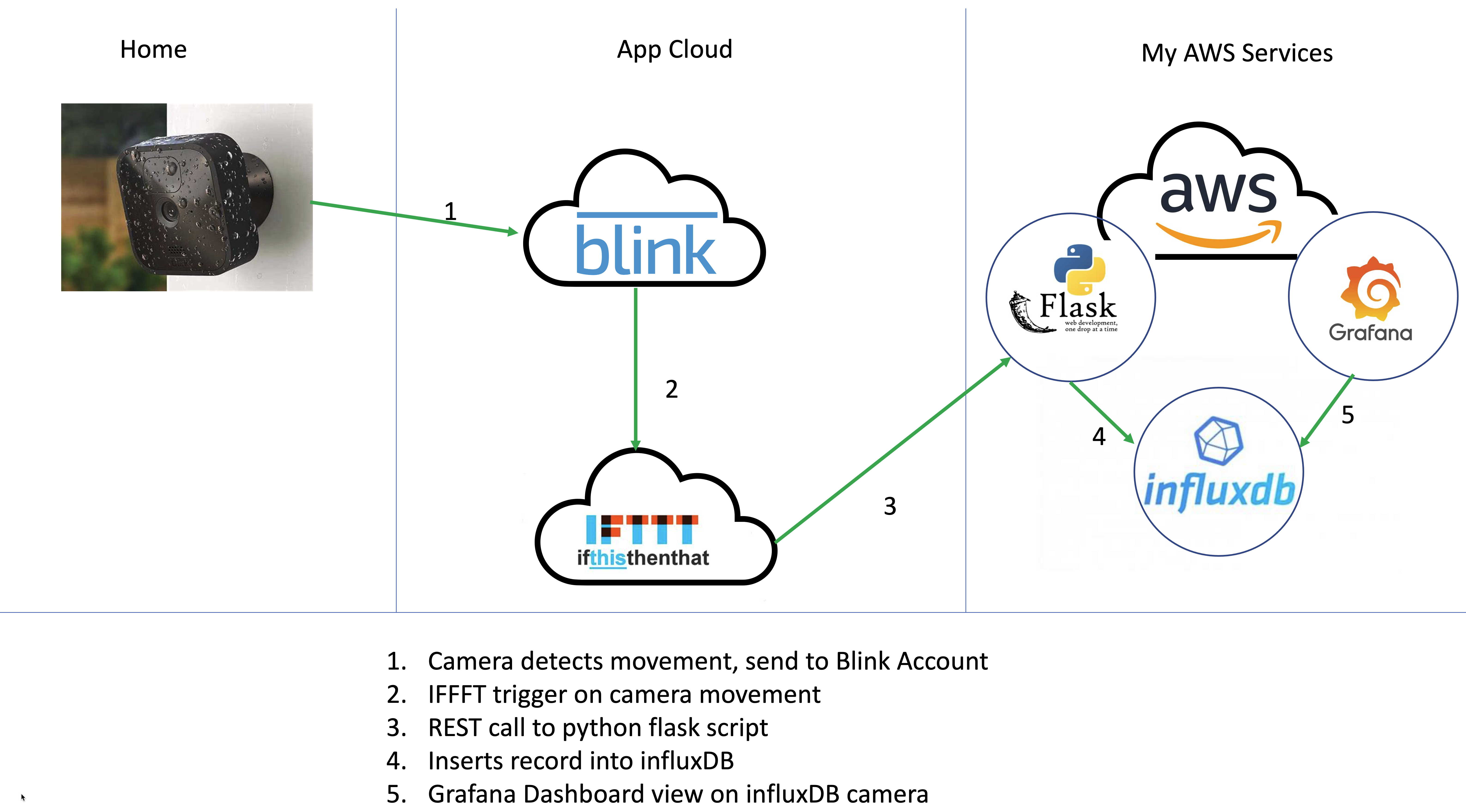
First, in IFFFT connect to your blink account and create a trigger to send a web request on motion detection on a particular camera.
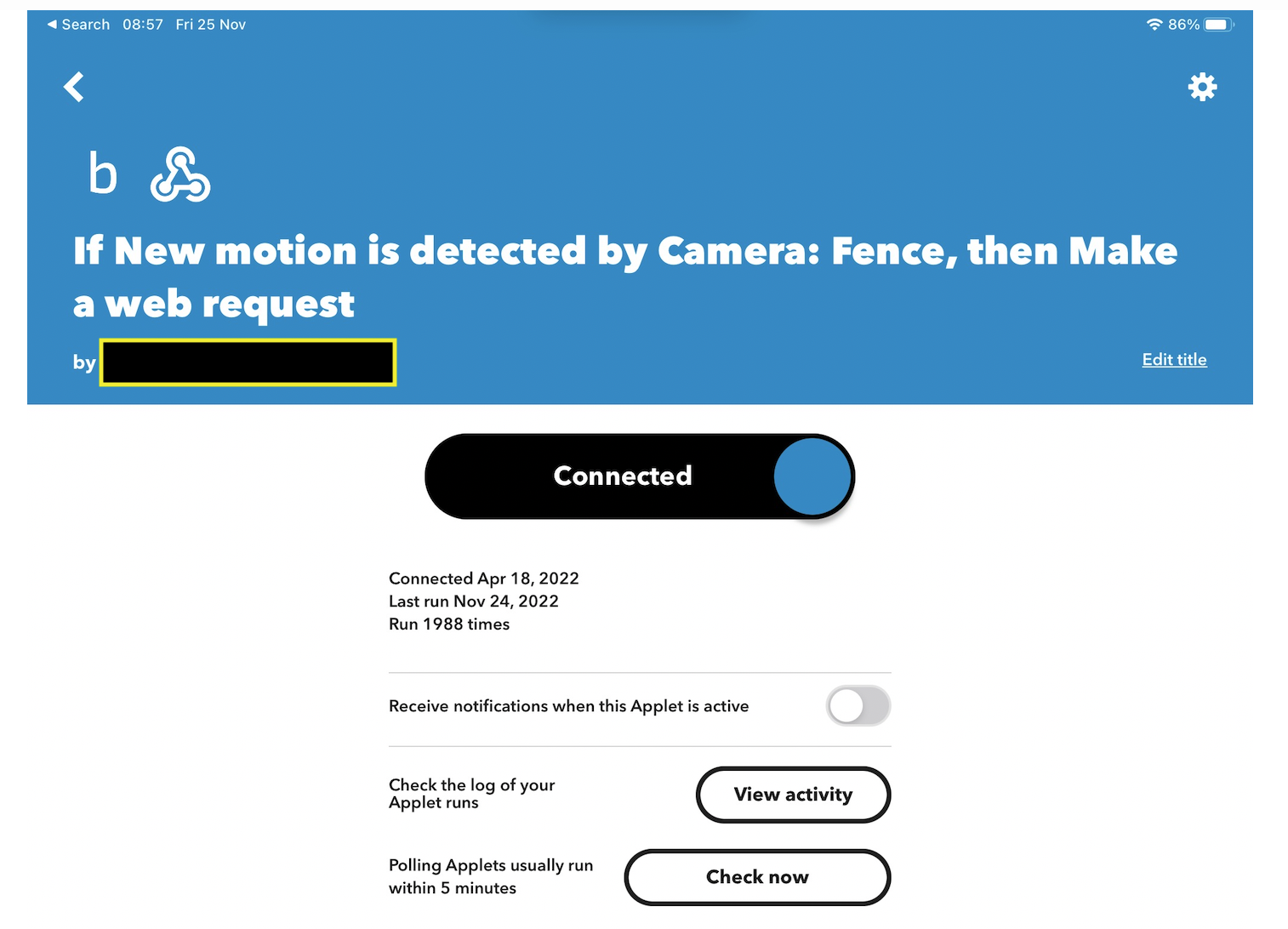
In the details section enter the URL and parameters to send the request (this will be the python/flask app) - e.g. http://my-aws-python-flask-app.com/test?camera=[CameraName]&time=[CreatedAt] where variables in square brackets [ ] are auto populated by the trigger at firing time.
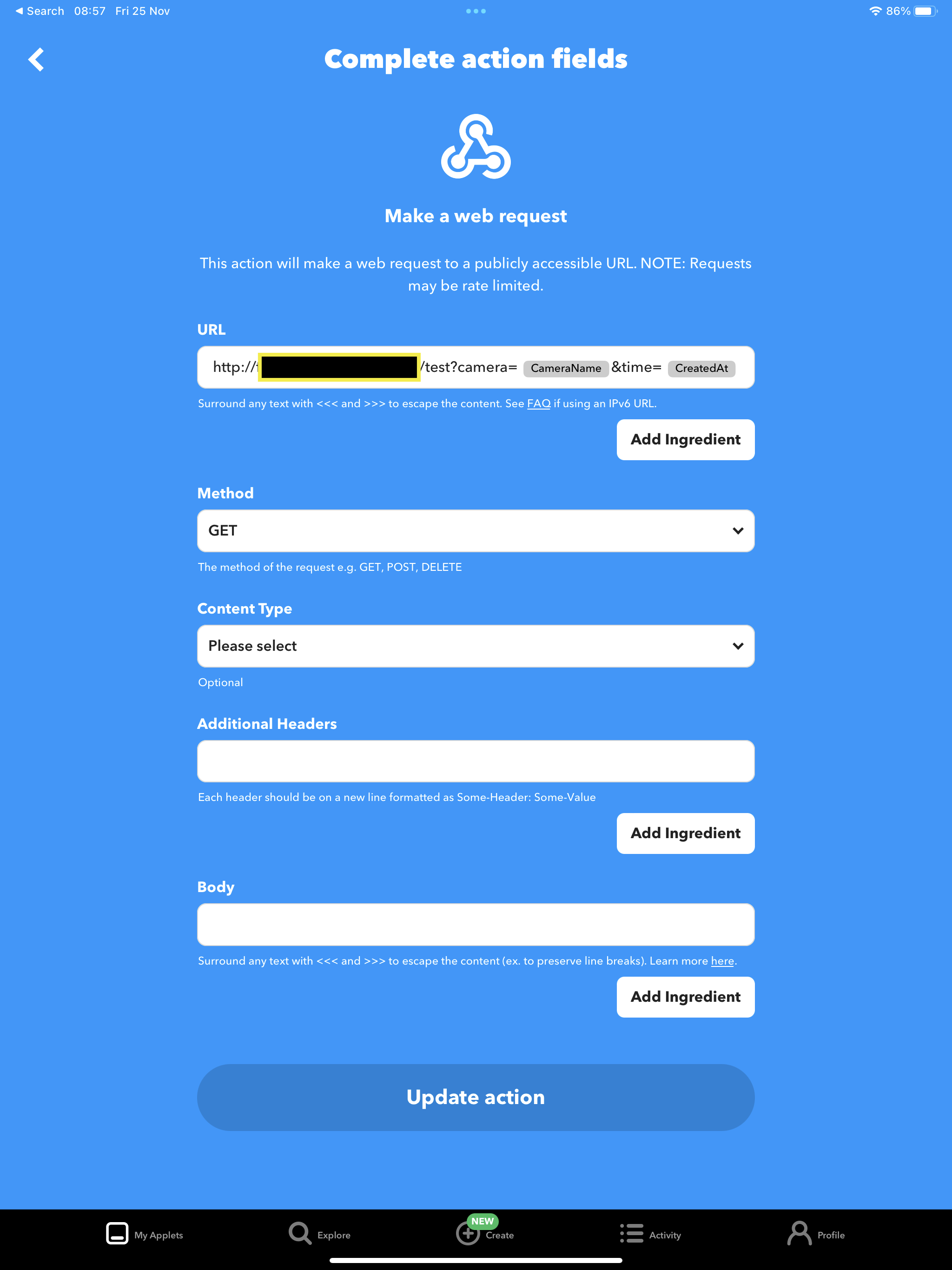
An example REST call is:
GET /test?camera=%20Fence&time=%20November%2024,%202022%20at%2010:42PM
The job of the python/flask app is to receive this REST call and translate the timestamp to a millisecond linux timestamp and insert it into influxDB in the correct format.
The main REST listener is defined as:
def test():
if 'camera' in request.args:
camera = request.args['camera']
print(camera)
if 'time' in request.args:
motionTime = request.args['time'].strip()
print(motionTime)
mtime = seg_date(motionTime)
user_data = camera + "|" + mtime
send_influx(camera,mtime)
return "OK"
Where the date conversion is:
def seg_date(dt_string):
import datetime
#example July 7, 2021 at 05:21PM
format = "%B %d, %Y at %I:%M%p"
passed_dt_object = datetime.datetime.strptime(dt_string, format)
timestamp = int(datetime.datetime(dt_object.year, dt_object.month, dt_object.day, dt_object.hour, dt_object.minute).timestamp())
print("Timestamp: ", str(timestamp)+"000000000")
return str(timestamp)+"000000000"
and then the influxDB insert is:
def send_influx(camera,mtime):
import requests
data = "motion,camera=%s value=1i %s" % (camera.strip(),mtime)
try:
r = requests.post("http://127.0.0.1:8086/write?db=blinkmotion", data=data)
except:
print("Error sending to influx")
return
Happy to provide more info on this.
Comments